Summary
Teleport is a communication abstraction layer. It helps deliver messages between application tiers or components without being tied to a specific networking technology.
Teleport allows building networked applications without writing networking codes
Conceptual example
As a simple example, assuming we have an order tracking system. At the first attempt, our application supports single user, single database. In essence, it is a standalone application.
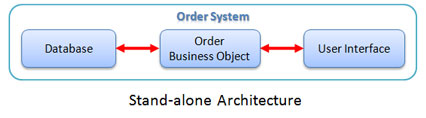
Notice the red arrows. They represent the communications between sub-components in our system. For simple standalone applications, they are typically just procedural calls.
To support multi-users, we will transform our simple application to client-server archiecture:
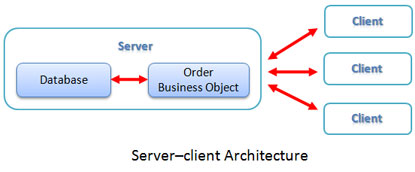
In this architecture, communication between server and client can be procedural calls or well-known services.
To support even more users, we need to scale up to multi-tier, service-based architecture:
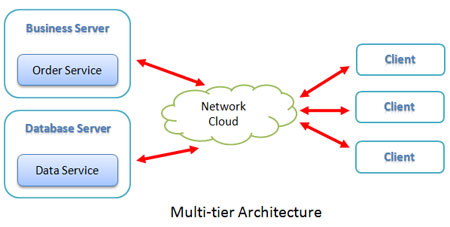
With the rising in popularity of SOA (service oriented architecture), message-based communication between application tiers or services is de facto standard.
The question is, what technology should we use for communication?
For .Net developers, there are quite many choices. The following are common ones:
- * Message-based (via Xml): web service, Soap, Xml RPC
- * Proprietary protocol: .Net Remoting, NamedPipe IPC
- * Do-it-yourself: TCP, UDP, file monitoring
The problem of choosing a communication protocol is the choosing itself. There are so many choices, none is satisfactory enough. Xml-based is too slow and requires not very friendly learning. Proprietary protocol is not compatible to other platform, or even worse, not compatible to its old versions. Low level protocols are simply too much hassle to work with.
Furthermore, transforming an application from stand-alone architecture to clien-server or multi-tier usually means re-developing almost everything from scratch.
Teleport technology saves us from the above problems by not having to choose anything. Developers do not need to choose any protocol of the above because they can switch the protocol quickly with a few line in configuration.
Not only the codes are protected from change, the developers are spared from learning specific protocols as well. No need to know how to config web services, no need to know how to properly invoke .Net remoting, and etc. They can use the same code again and again without having to know whether the service they need is local or remote.
* Allow applications to switch networking protocol without changing codes
* Allow applications to switch artchitecture without changing codes
* Free developers from learning networking protocol
* Independent from underlying networking protocol
* Independent from message packaging methodology
* 100% pure managed code
* CLS (Common Language Specification) 1.1 and POCO conformant
In this tutorial, we will develope codes for the previous Conceptual Example. Using Teleport, we can develope all three scenarios: standalone, client/server, and multi-tier architecture quickly with just a few changes in configuration codes.
Stand-alone bare-bone
Assuming we are buiding the standalone version, the main OrderService class is similar to this:
Figure 1: Declare OrderService
public class OrderService
{
public string PlaceOrder(Order value)
{
//process new order
//then return a confirmation tracking number
return "o1234";
}
}
The client (consumer) code which uses order service is similar to the following:
Figure 2a: Consume order service
private void btnNewOrder_Click(object sender, EventArgs e)
{
Order o = new Order();
string confirm = service.PlaceOrder(o);
}
Stand-alone with Teleport
To use Teleport, we make a few modifications to the above code.
First, the client code in Figure 2a will become:
Figure 2b: consume order service using Teleport
private void btnNewOrder_Click(object sender, EventArgs e)
{
Order o = new Order();
string confirm = Teleport.Request("Order System", "PlaceOrder"
, o) as string;
}
The above code instructs Teleport to invoke method PlaceOrder from gateway Order System (gateway is a unique address similar to URL to let Teleport know where to deliver our message to). As you can see, our method has not been changed much. We simply replaced a direct method call with an indirect call using Teleport.
Since the Teleport does not know of our Order Service yet, we will register the service to Teleport using the following codes:
Figure 3: register a gateway for order service
void RegisterGateways()
{
Teleport.AddGateway("Order System", new OrderService());
}
Since we are developing the stand-alone version of our Order System, we will put the RegisterGateways() method in the start of our application.
Client-Server & Multi-tier
To make our system become client-server or multi-tier architecture, we do not need to redesign the system or write any networking code.
We need to move the OrderService declaration (figure 1) and RegisterGateways (figure 3) to the server. The client code does not need those things anymore.
How will the messages be transmitted?
Depends on our needs, we may tell the Teleport to use .Net Remoting, web service, or even write our custom networking transporter. It does not matter what networking mechanism we use, our codes are still protected from change. The Teleport works transparently.
The following xml configurations will look intimidating at first. But please remember that we simply need to copy and paste them. The only thing we need to care about is our codes. Those precious codes are not changed, regardless of networking mechanism we use.
.Net Remoting
To use .Net Remoting, we will create a Teleport.xml configuration file as following:
Figure 4a: xml configuration for .Net Remoting
<Configurations>
<Teleport>
<Name>Remoting</Name>
<!--Name of the class type that will be used as the connector-->
<Connector>Thn.Communication.Teleporting.Connectors
.Remoting.ClientConnector</Connector>
<!--[Optional] name of the .dll file which contains the class-->
<ContainerFile>Thn.Communication.Teleporting.Connectors.
.Remoting.dll</ContainerFile>
<!--Connector specific parameters-->
<!--
Possible values of:
ChannelType: Tcp, Http
Formatter : Binary, Soap
-->
<Server>localhost</Server>
<Port>8080</Port>
<Uri>TeleportService</Uri>
<ChannelType>Tcp</ChannelType>
<Formatter>Binary</Formatter>
</Teleport>
</Configurations>
Web Service
To use Web Service, the configuration file will look like this:
Figure 4b: xml configuration for web service:
<Configurations>
<Teleport>
<Name>WebService</Name>
<!--Name of the class type that will be used as the connector-->
<Connector>Thn.Communication.Teleporting.Connectors
.WebService.WebServiceConnector</Connector>
<!--[Optional] name of the .dll file which contains the class-->
<ContainerFile>Thn.Communication.Teleporting.Connectors
.WebService.dll</ContainerFile>
<!--Connector specific parameters-->
<Url>http://localhost:31808/TeleportService/TeleportService.asmx</Url>
<!--[Optional] name of the teleporting method on web service-->
<Method>TeleportRequest</Method>
<!--[Optional] Xml namespace of the webservice (not the class namespace)-->
<Namespace>http://tempuri.org/</Namespace>
</Teleport>
</Configurations>
Custom Transport
To use custom networking solution, we need to tell Teleport where to find the connector. The following example demonstrate how to config Teleport for a loopback (no actual network) scenario:
Figure 4c: xml configuration for custom transport
<Configurations>
<Teleport>
<Name>Loopback</Name>
<!--Name of the class type that will be used as the connector-->
<Connector>Thn.Communication.Demo.Connectors
.Loopback.LoopbackConnector</Connector>
<!--[Optional] name of the .dll file which contains the class-->
<ContainerFile>Thn.Communication.Demo.Connectors
.Loopback.dll</ContainerFile>
</Teleport>
</Configurations>
The beauty of Teleport is the fact that it... does not do anything.
Teleport is designed on the assumption that SOA (service oriented architecture) application focus on messages (the content of business objects) rather than remote procedure invocation or data streaming. As the results, message - focused application does not need to deal with the hassle of networking like those such as online game systems. Since the SOA developers need not to deal with networking code, they should not have to do so. However, there are many existing networking technologies available, each suitable for specific scenarios. Consequently, the Teleport itself does not rely on any particular networking technology.
The Concept of Teleportation
For science-fiction fans, teleportation is well known. To others, teleportation means an object (human, cat, machine, etc.) can be at point A, then "teleported" (moved and appear instantly) at point B. A more interesting explanation can found here : http://www.howstuffworks.com/teleportation.htm
Back to our system, using Teleport, an arbitrary object (class) can be transferred from a client to a server. What makes Teleport interesting is that our message can be anything. It can be text, xml, object, whatever.
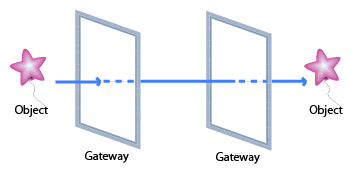
Given an object, send it in a gateway, we should get the exact object at the other gateway. We care about getting our object back exactly as it is, without paying attention to how it is transported.
Architecture
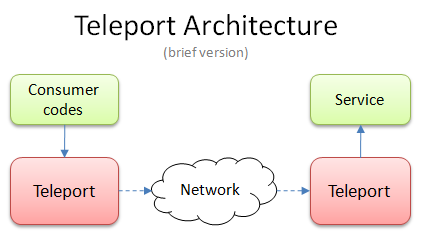
(click for more detailed diagram)
The Teleport mainly consists of 4 components: the Teleport itself, a communicator, and a codec. The codec is responsible for packing and unpacking message (serialize / deserialize arbitrary object(s) to / from common medium). The communicator is responsible for interfacing with the actual networking protocol. The Teleport itself is the orchestrator that consumer and service code interact with.
To put it in another way, let's take the mail delivery example. John Doe has a love letter. He wants to send it to Jane. After much thought invested in the letter, he wraps the letter in a cover and goes to the post office. An office clerk takes his mail, put it in a delivery box and send it away. The mail will not be transferred until a truck driver drives the mail to the other post office at the destination. Then, Jane will be informed of the incoming mail. She picks it up, unwrap the letter, reads it. The rest of the story depends on how much Jane likes John.
In terms of Teleport, John is the consumer (client) code, Jane is the service provider (providing... love service). The post office is the Teleport. Both John and Jane come to the post office to send and pickup a message. The communicator is the truck driver, who is responsible for physically deliver the message.
The interesting point in the story is the love letter. Notice how it is handled? Whatever John writes in the letter, Jane receives the exact message. Nobody else, the post office clerk, or the truck driver knows the content of the letter. As is the case of Teleport. The Teleport itself does not know anything about the object(s) it has to transmit. It does not know how to pack or unpack a message. It does not even know how to physically transmit a message. Hence, we coined the expression: "the beauty of Teleport is the fact that it does not do anything".
To be fair, Teleport or the post office, is responsible for orchestrating the whole process. This is the principle of "separation of intent" at work. Consumer needs not know about the communication. The service provider cares only for its service. The codec is in charge of packing and unpacking message. The communicator takes care of transmitting. The Teleport is the process manager.
This architecture encapsulates multiple layers at once. As the results, we achieve "coding networked application without networking codes" while still reserving the power to choose whatever networking technology best suits our needs.